Try this
// input data is a string of character 1s and 0s representing bits
string = "11110000111000110010";
// split the string in two at the middle
// this substring starts at character 1 and goes for half the length
firsthalf = Substr( string, 1, Length( string ) / 2 );
// this substring starts just beyond the previous and the
// implicit length goes to the end
secondhalf = Substr( string, 1 + Length( string ) / 2 );
// the 1 and 0 bits go one per column
ncols = Length( firsthalf );
// start a fresh table
dt = New Table( "example" );
// add columns wtih names like "c3" for each bit
For( i = 1, i <= ncols, i++, // the || operator joins two strings
dt << New Column( "c" || Char( i ) ) // i is a number, char makes a string
);
// make a helper function to add bits to a row in dt
adddata = Function( {bits}, // parameter to function
{i, c}, // local variables
dt << addrows( 1 ); // function knows to use dt
For( i = 1, i <= Length( bits ), i++,
c = Column( dt, "c" || Char( i ) ); // same as above
dt:c = Num( Substr( bits, i, 1 ) ); // put bit in column
);
);
// each call to adddata puts a row of bits in dt
adddata( firsthalf );
adddata( secondhalf );
adddata( "1010101010" );
adddata( "1111111111" );
adddata( "0000000000" );
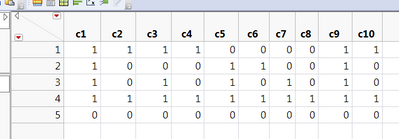
Craige