You could replace the HAVING clause by creating your condition in a second query, joining that into your original select statement, and then filtering the result. Here is what that might look like:
SELECT a.column-names
FROM table-name a
LEFT JOIN (SELECT condition-group-column, summary(condition-summarized-column) as condition
FROM table-name
GROUP BY condition-group-column) b
ON a.condition-column = b.condition-column
WHERE condition > 1;
You could test this in db-fiddle using this in the schema:
CREATE TABLE test (
id INT, val INT
);
INSERT INTO test (id, val) VALUES (1, 10);
INSERT INTO test (id, val) VALUES (2, 10);
INSERT INTO test (id, val) VALUES (3, 20);
INSERT INTO test (id, val) VALUES (4, 30);
INSERT INTO test (id, val) VALUES (5, 30);
and this in the select:
SELECT a.ID, a.val, b.tot
FROM test a
LEFT JOIN (SELECT val, count(id) as tot
FROM test
GROUP BY val) b
ON a.val = b.val
WHERE tot > 1;
which should look something like this:
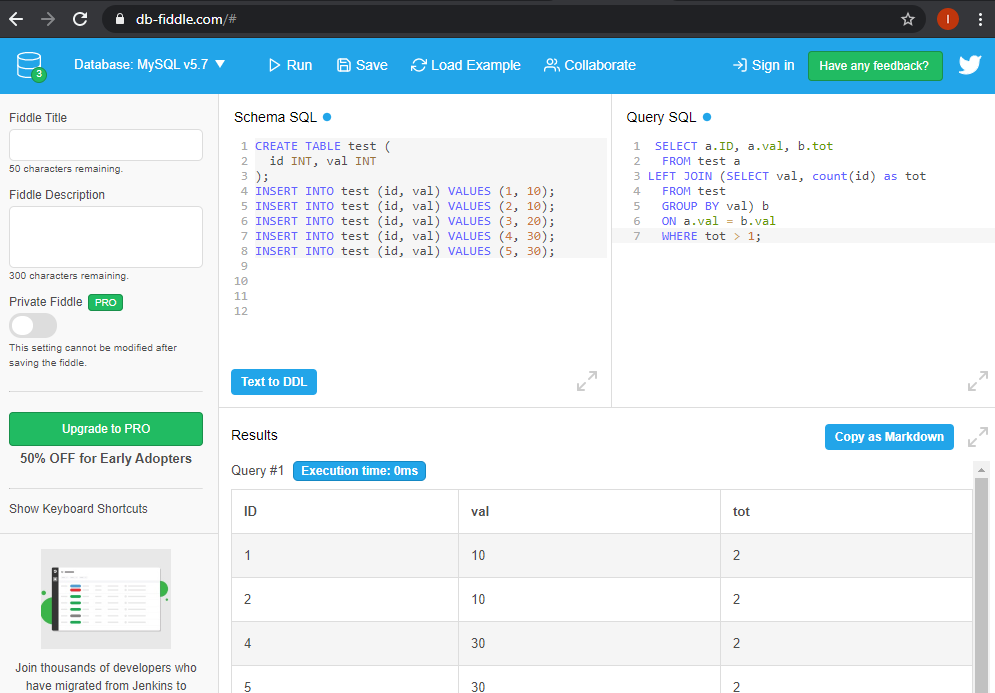