All,
I'd like to ask for an input on the best way to script a GB plot with multiple parameters.
Let's say I have a table like this:
New Table( "Untitled 140",
Add Rows( 12 ),
New Column( "Category",
Character,
"Nominal",
Set Values( {"A", "A", "A", "B", "B", "B", "C", "C", "C", "D", "D", "D"} )
),
New Column( "Value1",
Numeric,
"Continuous",
Format( "Best", 12 ),
Set Values( [1, 4, 2, 3, 6, 2, 3, 5, 4, 1, 3, 6] )
),
New Column( "Value2",
Numeric,
"Continuous",
Format( "Best", 12 ),
Set Values( [6, 3, 5, 2, 8, 6, 5, 2, 6, 3, 5, 4] )
)
)
And I want to script a plot like this:
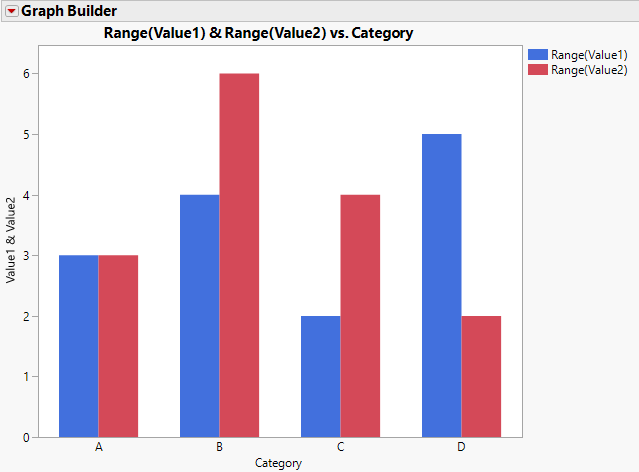
where the autogenerated script looks like this:
Graph Builder(
Size( 522, 450 ),
Show Control Panel( 0 ),
Variables( X( :Category ), Y( :Value1 ), Y( :Value2, Position( 1 ) ) ),
Elements( Bar( X, Y( 1 ), Y( 2 ), Legend( 4 ), Summary Statistic( "Range" ) ) )
)
;
with the catch that there is an arbitrary number of Y columns and the names are also arbitrary.
For plots like Variability plot I would something like this:
parameters = {:"Value1"n, :"Value2"n};
yExpr = Expr( Y() );
For Each( {value, index}, parameters, Insert Into( yExpr, Name Expr( value ), 1 ) );
Eval(
Substitute(
Expr(
Variability Chart( placeholder, X( :Category ), Std Dev Chart( 0 ) )
),
Expr( placeholder ), Name Expr( yExpr )
)
);
where I receive the names of the columns as a list and then construct a Y expression and then put it into the script for the chart. It would give me a list of variability plots, but that's OK with me.
If I want to take the same route in case of the GB it looks like I will have to construct two expressions:
Y( :Value1 ), Y( :Value2, Position( 1 ) )
and
Elements( Bar( X, Y( 1 ), Y( 2 ), Legend( 4 ), Summary Statistic( "Range" ) ) )
Which is essentially the whole script.
My question: Is there a better way rather than making bunch of substitutions with expressions to assemble those two lines?
Thanks!