I have added a few lines of code that will eliminate the duplicate combinations. In addition, I would suggest that you look into
Tables=>Join
at the Cartesian join capability.
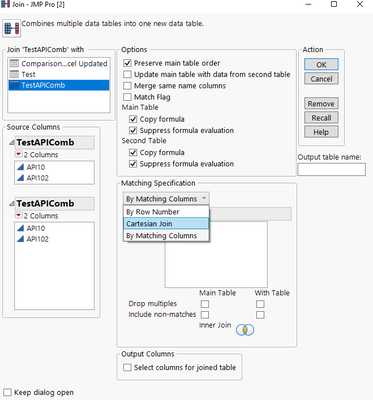
It produces the all possible combinations much faster than JSL code can do it.
Names Default To Here( 1 );
New Table( "Test",
Add Rows( 5 ),
New Column( "API 10", Numeric, "Continuous", Format( "Best", 15 ), Set Values( [4238939261, 4238939263, 4238939262, 4238939328, 4238939329] ) ),
);
Test = Data Table( "Test" );
APIs = Test:API 10 << get values;
working = New Table( "TestAPIComb", New Column( "API10" ), New Column( "API102" ), add rows( N Items( APIs ) ^ 2 ) );
APIs2 = Test:API 10 << get values;
theRow = 0;
For( APICNT = 1, APICNT <= N Items( APIs ), APICNT++,
For( API2CNT = 1, API2CNT <= N Items( APIs2 ), API2CNT++,
theRow++;
:API10[theRow] = APIs[APICNT];
:API102[theRow] = APIs[API2CNT];
)
);
dt = Data Table( "TestAPIcomb" );
// Arange data so order is the same for all rows
For( i = 1, i <= N Rows( dt ), i++,
If( :API102[i] > :API10[i],
hold = :API10[i];
:API10[i] = :API102[i];
:API102[i] = hold;
)
);
// Find the duplicates
dt << Select Duplicate Rows( :API10, :API102 );
// Delete the duplicates
dt << delete rows;
Jim