Problem
In many situations, when you are using a slider box to control a numeric variable, you also want a editable number display to display the value of the variable, and that allows you to manually set the value of the variable.
The current way to do this is to create two display objects: a Number Edit Box() and a Slider Box(). You have to code this in such a way that they are connected. In addition, the way the number edit box interacts with changes to manually entered values (using <<Set Function()) is different than the way the Slider Box works (providing an expression as an argument to the display box that runs evertime the slider is moved).
I often find myself having to take a lot of time reading the scripting guide on how to create these objects and how to connect them. (I wish there were an built in JMP display object like this)
Solution
I have created a function SliderNumberEditBox() that creates an evaluated expression that creates both display boxes together. These display boxes will be connected as described above. It takes as arguments character strings that define the references to the Number Edit Box, the initial value for the Number Edit Box, the reference to the Slider Box, the upper and lower range for the slider box, the variable that is controlled by the slider box, and optionally, the expression you want to run when the Number Edit Box is updated manually, and the expression you want to run when the Slider Box is changed. (note that the expressions must be defined as character strings, and the appropriate escape sequences must be used for reserved characters that you want quoted inside the string).
SliderNumberEditBox = Function( {nebref, nebinitval, sbref, sblower, sbupper, sbvar, nebFunction = "", sbFunction = ""},
Eval(
Parse(
"H List Box(" || nebref || " = Number Edit Box(" || nebinitval || ",
<<Set Function(
Function( {this}," || sbref ||
" << set( this << get );" || nebFunction || "
)
)
)," || sbref || "= Slider Box(" || sblower || "," || sbupper || ","
|| sbvar || "," || nebref || " << Set( " || sbvar || " );" || sbFunction || "
)
)
"
)
)
);
The function uses string concatenation to create the entire string version of the expression for the display boxes, and then parses the string into an expression and returns the evaluated expression.
Syntax
SliderNumberEditBox(string for the reference to the number edit box, string for the initial value of the number edit box, string for the reference to the slider box, string for the lower range of the slider, string for the upper range of the slider, string for the variable controlled by the slider, <string representation of the expresssion you want to evaluate when the number edit box is manually changed>, <string representation of the expression you want to evaluate when the slider box is changed>)
k = 1;
SliderNumberEditBox(
"_neb1",
Char( k ),
"_sb1",
"0",
"2",
"k")
returns the evaluated expression below
H List Box(
_neb1 = Number Edit Box(
0.5,
<<Set Function( Function( {this}, _sb1 << set( this << get ) ) )
),
_sb1 = Slider Box( 0, 2, k, _neb1 << Set( k ) )
)
Example
SliderNumberEditBox = Function( {nebref, nebinitval, sbref, sblower, sbupper, sbvar, nebFunction = "", sbFunction = ""},
Eval(
Parse(
"H List Box(" || nebref || " = Number Edit Box(" || nebinitval || ",
<<Set Function(
Function( {this}," || sbref ||
" << set( this << get );" || nebFunction || "
)
)
)," || sbref || "= Slider Box(" || sblower || "," || sbupper || ","
|| sbvar || "," || nebref || " << Set( " || sbvar || " );" || sbFunction || "
)
)
"
)
)
);
k = 1;
_nw = New Window( "Slider Number Edit Box",
_vlb = V List Box(
SliderNumberEditBox(
"_neb1",
Char( k ),
"_sb1",
"0",
"2",
"k",
"
_tb << delete;
_vlb << Append(
_tb=Text Box(\!"The number is \!" || char(k))
)",
"
_tb << delete;
_vlb << Append(
_tb=Text Box(\!"The number is \!" || char(k))
)"
),
_tb = Text Box( "The number is " || Char( k ) )
)
);
creates a window that looks like this (I changed the slider box to a different value)
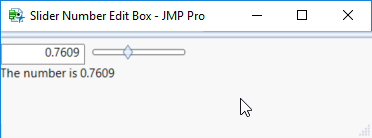