The code below should allow you to dynamically profile any saved formula column that involves only one input. In the case of 'Big Class' and a quartic model, it gives:
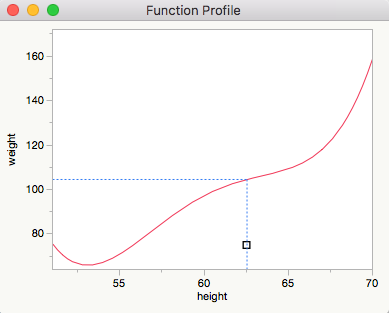
It's more or less untested.
NamesDefaultToHere(1);
// Function to find leaves in formula that are columns in the table
// See: https://community.jmp.com/blogs/Uncharted/2015/04/03/head-arg-recurse-formula"
// Thanks to Craige Hales
findColumns =
Function( {frm, cols},
{h = Head( frm ), n = N Arg( frm ), i, result = {}}, // local variables
If( n == 0, // head node has no children, it is a leaf
If( Contains( cols, Name Expr( h ) ), // is the leaf in the list of columns?
Insert Into( result, Name Expr( h ) ); // yes -> add to result
)
,
For( i = 1, i <= n, i++, // not a leaf, get children's results
Insert Into( result, Recurse( Arg( frm, i ), cols ) )
)
);
result; // return the result
);
// Open a table to play with, and add a formula column to it
dt = Open("$SAMPLE_DATA/Big Class.jmp");
fCol = dt << New Column( "Predicted weight",
Formula(-127 + 4 * :height),
Set Property("Predicting", {:weight, Creator( "Bivariate" ), Model Name( "Polynomial Fit Degree=3" )})
);
// Recover the required information
f = fCol << getFormula;
xColList = findColumns(NameExpr(f), dt << getColumnNames);
yCol = fCol << getProperty("Predicting");
yCol = yCol[1];
// Subsequent code will only work with one x Column
xCol = Expr(xColList[1]);
for(c=1, c<=NItems(xColList), c++, Print(c); if(xColList[c] != xCol, Print("Only one x Column allowed."); Beep(); Throw()));
// Reparameterise the formula for later use in the 'GraphBox()'
SubstituteInto(f, xCol, Expr(x));
// Set the initial 'VLine()' value to the mean of the x values
xVals = xCol << getValues;
x = Mean(xVals);
// Get 'reasonable' ranges for the axes
xMin = Min(xVals);
xMax = Max(xVals);
yMin = Min(yCol << getValues);
yMax = Max(yCol << getValues);
// Get the names of the columns involved
xColName = xCol << getName;
yColName = yCol << getName;
// Plot the graph
NewWindow("Function Profile",
gb = GraphBox("Simple Profiler", XScale(xMin, xMax), YScale(yMin, yMax), XName(xColName), yName(yColName),
// Plot the function
PenColor("Red"); YFunction(f, x);
// Handle to drag the 'VLine()' and update display
Handle(x, yMin + (yMax-yMin)/10, gb << reShow, Empty());
// Get the function value for the current x value
yy = Eval(Substitute(NameExpr(f), Expr(x), x));
// Plot the lines at the new places
PenColor("Blue"); LineStyle("Dotted");
VLine(x, yMin, yy);
HLine(xMin, x, yy);
);
);