Given the scenarios you specified, the script below, generates the 3 cases
A exists in Column A
B exists in Column B
Neither A or B exist
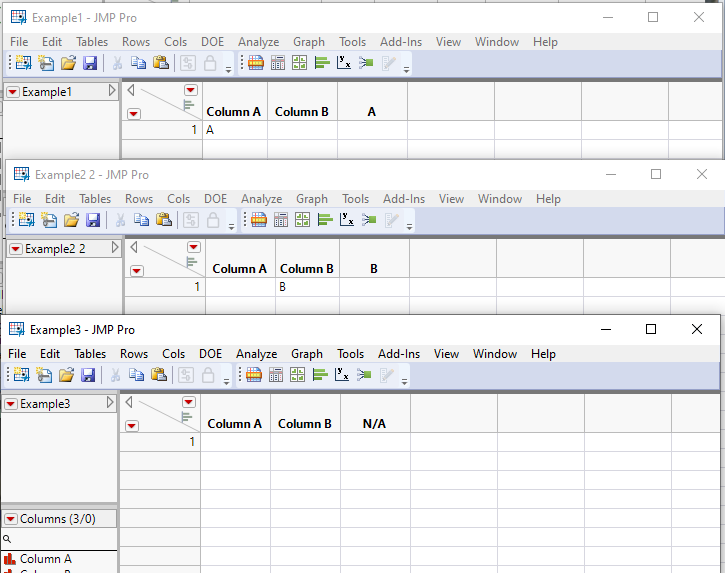
Names Default To Here( 1 );
A = Function( {},
Show( "Function A" )
);
B = Function( {},
Show( "Function B" )
);
C = Function( {},
Show( "Function C" )
);
D = Function( {},
Show( "Function D" )
);
EE = Function( {},
Show( "Function EE" )
);
F = Function( {},
Show( "Function F" )
);
// First Example Column A has the value A
dt1 = New Table( "Example1",
Add Rows( 1 ),
New Column( "Column A", Character, "Nominal", Set Values( {"A"} ) ),
New Column( "Column B", Character, "Nominal", Set Values( {""} ) )
);
Wait( 5 );
If(
:Column A[1] == "A",
dt1 << New Column( "A", character, nominal );
A();
B();
C();,
:Column B[1] == "B", dt1 << New Column( "B", character, nominal );
D();
EE();
F();
,
dt1 << New Column( "N/A", character, nominal )
);
// Second Example Column B has the value B
dt2 = New Table( "Example2",
Add Rows( 1 ),
New Column( "Column A", Character, "Nominal", Set Values( {""} ) ),
New Column( "Column B", Character, "Nominal", Set Values( {"B"} ) )
);
Wait( 5 );
If(
:Column A[1] == "A",
dt2 << New Column( "A", character, nominal );
A();
B();
C();,
:Column B[1] == "B", dt2 << New Column( "B", character, nominal );
D();
EE();
F();
,
dt2 << New Column( "N/A", character, nominal )
);
// Third Example Neither column A has and A nor Column B has a B
dt3 = New Table( "Example3",
Add Rows( 1 ),
New Column( "Column A", Character, "Nominal", Set Values( {""} ) ),
New Column( "Column B", Character, "Nominal", Set Values( {""} ) )
);
Wait( 5 );
If(
dt3:Column A[1] == "A",
dt3 << New Column( "A", character, nominal );
A();
B();
C();,
dt3:Column B[1] == "B", dt3 << New Column( "B", character, nominal );
D();
EE();
F();
,
dt3 << New Column( "N/A", character, nominal )
);
It is my guess, that this is not what you want
Jim